Workflow Parameter Types#
This article describes the different ui widgets available through the @parameter
decorator. These widgets will show up in the request form to allow a user to set the value of workflow parameters.
Text (default)#
Defining the parameter as a string will result in the text field on the request module. There is no need to specify a uiWidget.

from artificial.workflows.decorators import parameter, workflow
@parameter('text_field', {'required': True, 'uiTitle': 'Text Field'})
@workflow("Text Field Workflow",
"wf_text",
"lab_e9ed9a16-1888-4050-a51d-9584caa0150f")
async def wf_func_name(text_field: str) -> None:
pass
Numeric#
Defining the parameter as an integer or float will result in the numeric field on the request module. There is no need to define a uiWidget.
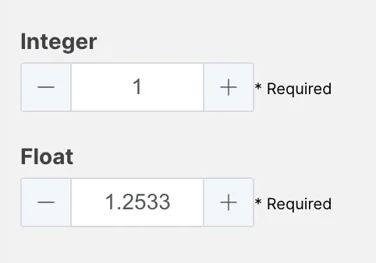
from artificial.workflows.decorators import parameter, workflow
@parameter('integer', {'required': True, 'uiTitle': 'Integer'})
@parameter('float', {'required': True, 'uiTitle': 'Float'})
@workflow("Numeric Field Example",
"wf_numeric",
"lab_e9ed9a16-1888-4050-a51d-9584caa0150f")
async def wf_func_name(integer: int, float: float) -> None:
pass
Array#
To create an array input, you do not need to define the UI widget. Instead, define the parameter as a List[str]
. Then, you can list your strings with commas.

from typing import List
from artificial.workflows.decorators import parameter, workflow
@parameter('array', {'required': False, 'uiTitle': 'Array'})
@workflow("Array Workflow", "wf_array", "lab_e9ed9a16-1888-4050-a51d-9584caa0150f")
async def wf_func_name(array: List[str]) -> None:
pass
File Upload#
To create a file upload option in the request module, the UI widget must be defined as 'file'
in the parameter decorator.
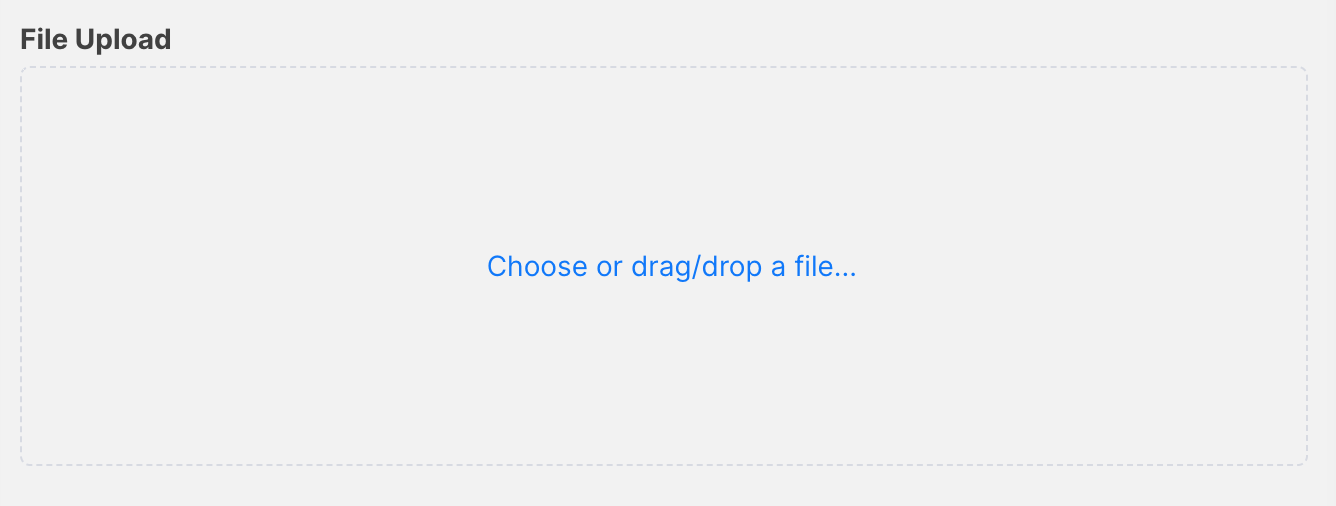
from typing import List
from artificial.workflows.decorators import parameter, workflow
@parameter('array', {'required': False, 'uiTitle': 'Array', 'uiWidget': 'file'})
@workflow("Array Workflow", "wf_array", "lab_e9ed9a16-1888-4050-a51d-9584caa0150f")
async def wf_func_name(array: List[str]) -> None:
pass
Checkbox#
By default, when a parameter argument is typed as a boolean, it will appear as a checkbox so no UI widget needs to be defined.
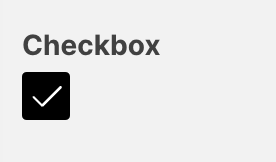
from artificial.workflows.decorators import parameter, workflow
@parameter('checkbox', {'required': False, 'uiTitle': "Checkbox"})
@workflow("Checkbox Workflow",
"wf_checkbox",
"lab_e9ed9a16-1888-4050-a51d-9584caa0150f")
async def wf_func_name(checkbox: bool) -> None:
pass
Switch#
By default, a boolean is a checkbox so to create a switch/toggle, you must specify the UI widget in the parameter function.
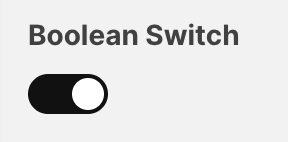
from artificial.workflows.decorators import parameter, workflow
@parameter('boolean_switch',
{'required': False, 'uiTitle': "Boolean Switch", 'uiWidget': 'switch'})
@workflow("Switch Workflow",
"wf_switch",
"lab_e9ed9a16-1888-4050-a51d-9584caa0150f")
async def wf_func_name(boolean_switch: bool) -> None:
pass
Select#
To create a drop-down selection field in the request module, the UI widget does not need to be defined. Instead, defining the workflow function argument type as EnumOptions
is sufficient.
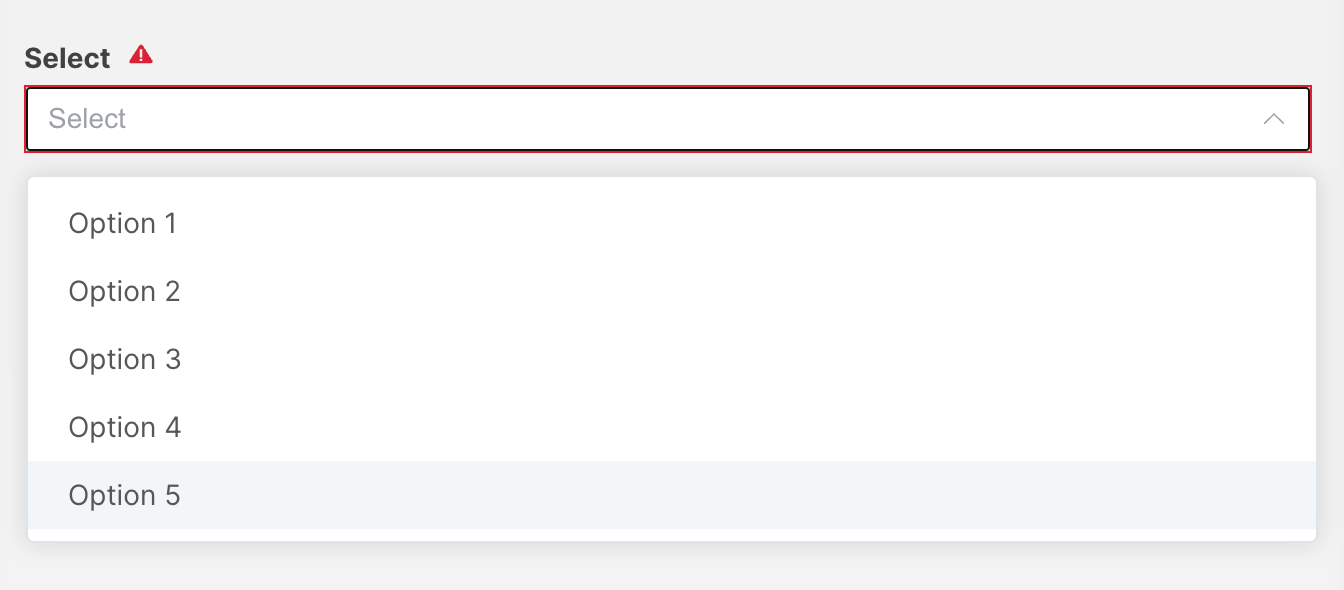
from enum import Enum
from artificial.workflows.decorators import parameter, workflow
class EnumOptions(Enum):
_1: str = 'Option 1'
_2: str = 'Option 2'
_3: str = 'Option 3'
_4: str = 'Option 4'
_5: str = 'Option 5'
@parameter('select', {'required': False, 'uiTitle': "Select"})
@workflow("Select Workflow", "wf_select", "lab_e9ed9a16-1888-4050-a51d-9584caa0150f")
async def wf_func_name(select: EnumOptions) -> None:
pass
Table#
You can create a table with set column names by defining the UI widget as 'table'
.
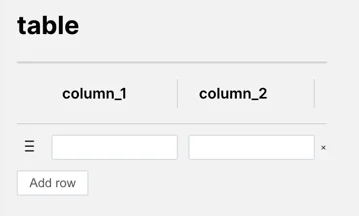
from dataclasses import dataclass
from typing import List
from artificial.workflows.decorators import parameter, workflow
@dataclass
class Table():
column_1: int
column_2: float
@parameter('table', {'required': False, 'uiTitle': 'Table', 'uiWidget': 'table'})
@workflow("Table Workflow", "wf_table", "lab_e9ed9a16-1888-4050-a51d-9584caa0150f")
async def wf_func_name(table: List[Table]) -> None:
pass